5 Proven Tips for Saving UserForm Data in Excel VBA

Excel's Visual Basic for Applications (VBA) provides a powerful platform for automating tasks, including handling UserForm data. Whether you're a beginner or an experienced VBA programmer, understanding how to save UserForm data effectively is crucial. In this detailed guide, we'll walk through five proven tips to ensure your UserForm data is saved efficiently and securely.
1. Define and Initialize Your Data Structures
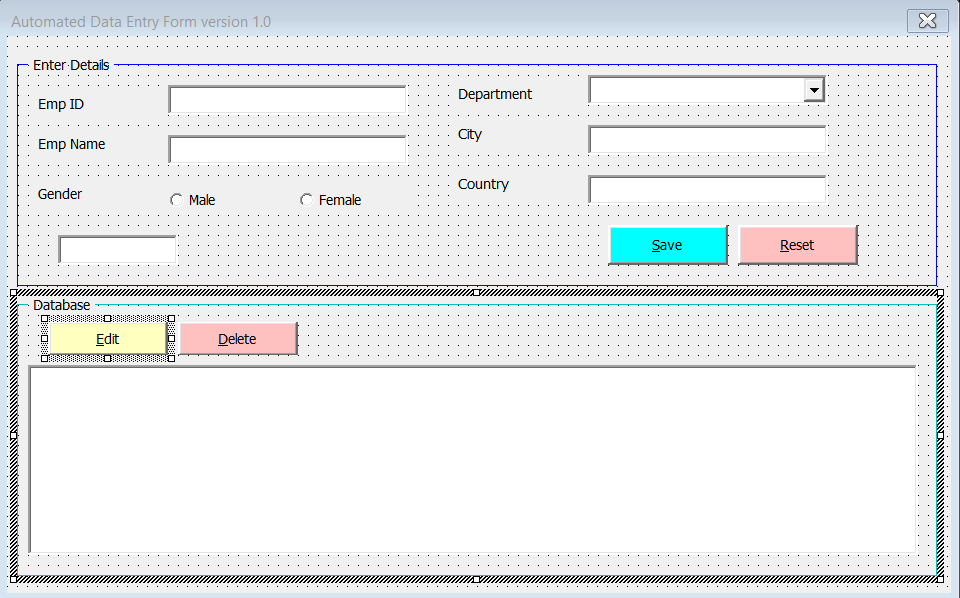
Before you start handling user input, it’s essential to define where this data will be stored. Using arrays or custom types can streamline data management:
- Arrays: Use when you need to store multiple entries of the same type.
- Custom Types: For grouping related data elements, making code more readable.
To initialize data:
Dim Data() As Variant
ReDim Data(1 To 1, 1 To 5) ' 2D array for storing up to 5 pieces of user data
💡 Note: Always dimension your arrays before filling them with data to avoid run-time errors.
2. Validate Data Before Saving

Before saving any data from your UserForm, it’s wise to validate user inputs for correctness and completeness. Here are some validation techniques:
- Range Checks: Ensure the data falls within the expected range.
- Pattern Matching: Use regular expressions or simple string functions to match data against expected patterns.
- Existence Checks: Verify that required fields are not left empty.
' Example validation function
Function IsValidData() As Boolean
If Len(Me.txtName.Value) = 0 Then
MsgBox "Please enter a name."
IsValidData = False
Else
IsValidData = True
End If
End Function
3. Use Transactions for Data Integrity

Data integrity is paramount when dealing with Excel. Use VBA’s transaction-like features to ensure atomicity:
- Begin Transaction: Start by disabling the worksheet's change events to prevent data alteration during the save operation.
- Save Data: Perform the save operation.
- End Transaction: Re-enable the worksheet's events if the save was successful, or revert changes if not.
' Turn off screen updating and events for transaction-like behavior
Application.ScreenUpdating = False
Application.EnableEvents = False
' Your data saving code here
If Success Then
Application.EnableEvents = True
Else
'Revert any changes made
MsgBox "Error saving data. Please try again."
End If
Application.ScreenUpdating = True
4. Implement Proper Error Handling

Errors in saving data can lead to data loss or corruption. Incorporate error handling to gracefully manage issues:
- Use the
On Error
statement to redirect to error handling routines. - Employ
Resume Next
to skip past errors and continue with the save process.
On Error GoTo ErrorHandler
' Code for saving data
Exit Sub
ErrorHandler:
MsgBox "An error occurred: " & Err.Description
Resume Next
💡 Note: Ensure that after handling an error, you either resume the next operation or exit the subroutine properly to avoid infinite loops.
5. Secure the Data with Passwords and Permissions

Protecting user data is vital. Here are some security measures:
- Worksheet Protection: Use passwords to restrict access to worksheets.
- Data Encryption: Consider encrypting sensitive data or storing it in a separate secure file.
- User Permissions: Limit what users can do with the data through custom VBA interfaces.
' Protect Worksheet
Me.Unprotect "Password"
' Modify data
Me.Protect "Password"
To wrap up, mastering the handling and saving of UserForm data in Excel VBA requires a strategic approach to data structure initialization, validation, integrity maintenance through transactions, error handling, and security. By following these proven tips, you can enhance the reliability and efficiency of your VBA applications, making sure that user data is managed with the highest standards of care. Whether you are a beginner or an advanced VBA user, these principles will aid in developing robust and user-friendly applications that uphold data integrity and user confidence.
What happens if I don’t validate user input in VBA?

+
Without validation, your application might save incorrect, incomplete, or harmful data, leading to errors, data corruption, or security vulnerabilities.
Can I use VBA to encrypt data in Excel?

+
Yes, VBA can encrypt data, either by calling out to encryption libraries or by using simple algorithms like XOR. However, remember that Excel itself is not designed for storing highly secure data.
How can I restore data if something goes wrong during saving?
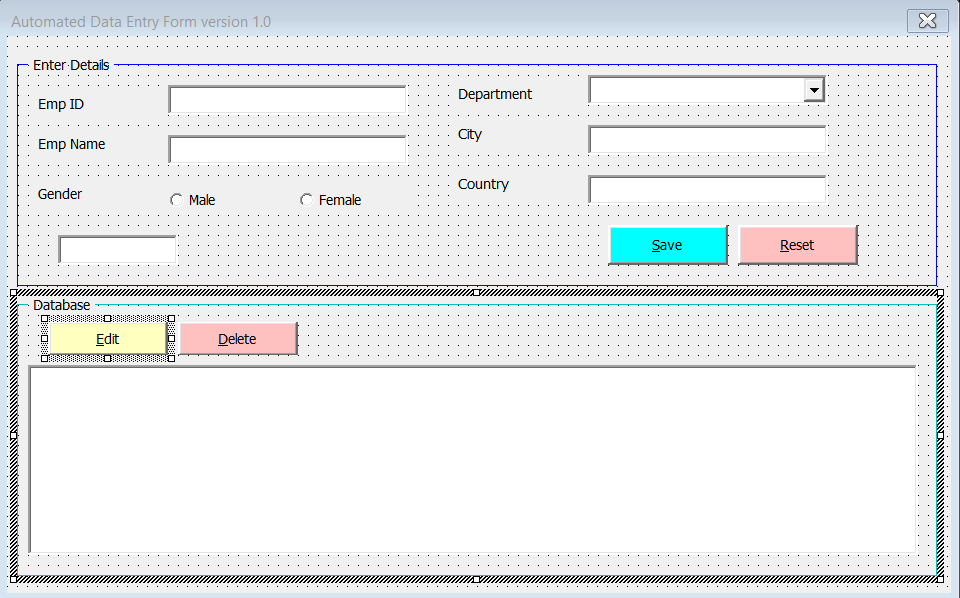
+
Use transaction-like structures in VBA where you can revert changes if an error occurs during the save operation, or consider saving data temporarily to a different sheet or file and then committing changes if all goes well.
Is it necessary to disable events in VBA for saving data?

+
Yes, disabling events during a save operation helps to prevent unintended side effects like worksheet change events firing unnecessarily, ensuring data integrity.