Master VBA: Selecting and Using the Active Worksheet Easily

In the vast realm of Microsoft Excel, VBA (Visual Basic for Applications) stands out as a powerful tool for automating tasks, manipulating data, and enhancing productivity. For those delving into Excel automation, understanding how to select and manipulate the active worksheet is fundamental. This blog post aims to guide you through the intricacies of working with the active worksheet in VBA, ensuring you can harness Excel's full potential for your data management needs.
Understanding Active Worksheet in VBA

Before diving into the how-to, it’s crucial to understand what an active worksheet means. In VBA, the active worksheet refers to the worksheet currently displayed in the Excel application, where all actions or inputs from the user are directed. It’s the sheet you’re working on at any given moment, and it can be manipulated through VBA coding to automate repetitive tasks or to perform complex data operations.
Selecting the Active Worksheet

To interact with the active worksheet, you first need to know how to reference it:
ActiveSheet
- This is the VBA keyword used to refer to the currently active worksheet. You can use it in your code to perform actions on this sheet.ThisWorkbook
- While not directly related to the active worksheet, it’s helpful to know this refers to the workbook in which your macro resides.
🔍 Note: Remember, ActiveSheet
will change if the user switches sheets manually or through your code.
Common Operations on the Active Sheet

Here are some common operations you can perform:
- Naming - Change the name of the active sheet.
- Navigation - Move between sheets or scroll through the active sheet's content.
- Selecting Ranges - Work with ranges of cells for data manipulation.
- Inserting or Deleting Sheets - Manage the workbook structure.
- Formatting - Apply formatting options like bold, color, and borders.
- Formulas and Functions - Add or modify formulas in cells or ranges.
Examples of VBA Code

Let’s delve into some practical VBA code examples for selecting and manipulating the active worksheet:
Naming the Active Worksheet
ActiveSheet.Name = “Dashboard”
Selecting a Range in the Active Worksheet
ActiveSheet.Range(“A1:B10”).Select
Adding a New Worksheet and Setting it as Active
ThisWorkbook.Sheets.Add(After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count)).Activate
Deleting the Active Sheet
Application.DisplayAlerts = False
ActiveSheet.Delete
Application.DisplayAlerts = True
Applying Formatting
With ActiveSheet
.Range(“A1:A10”).Font.Bold = True
.Range(“A1:A10”).Interior.Color = RGB(255, 255, 0) ‘ Yellow
End With
⚠️ Note: Always save your workbook before running scripts that delete sheets, as this action cannot be undone.
Advanced Techniques

Dynamic Reference to ActiveSheet
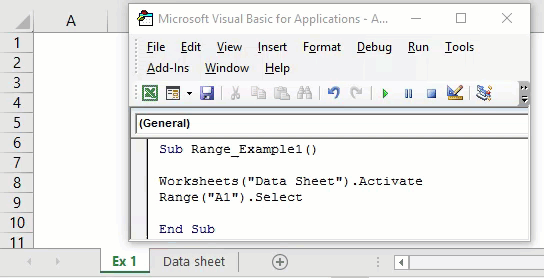
When you’re dealing with multiple workbooks or sheets, it’s often useful to store references dynamically:
Dim ws As Worksheet
Set ws = ActiveSheet
ws.Name = “Dynamic_Sheet_Name”
Handling Events

VBA can react to events in Excel, like when a sheet is activated or modified:
Private Sub Workbook_SheetActivate(ByVal Sh As Object)
MsgBox “You have activated ” & Sh.Name
End Sub
This event can be placed in the Workbook module to trigger a message box each time a different sheet is activated.
Iterating Through Worksheets

If you need to perform actions on multiple sheets, you might consider:
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If ws.Name = ActiveSheet.Name Then
‘do something specific to the active sheet
Else
‘do something for all other sheets
End If
Next ws
Best Practices for Working with ActiveSheet

- Avoid Overuse: While it’s easy to use
ActiveSheet
, overusing it can lead to issues if sheets are changed manually during macro execution. - Use Explicit References: Where possible, explicitly reference sheets by name or index to avoid unintended interactions with the wrong sheet.
- Error Handling: Incorporate error handling to manage situations where the active sheet might not exist or is protected.
- User Interface: Provide feedback or user prompts to minimize confusion when sheets are manipulated programmatically.
💡 Note: Use Option Explicit
at the start of your VBA modules to catch undeclared variables, which can help prevent errors related to sheet references.
Conclusion

Navigating and manipulating the active worksheet in VBA can transform your workflow in Excel, allowing for efficient data management and automation. Understanding how to reference, select, and modify the active worksheet empowers you to execute complex tasks with ease. By following the practices and tips outlined, you’ll be better equipped to develop robust, error-free VBA code that enhances productivity and minimizes manual intervention in your Excel workbooks. Whether it’s for simple renaming tasks, formatting large data sets, or complex data operations, mastering VBA’s handling of the active worksheet is a valuable skill in any data professional’s toolkit.
What is the difference between ActiveSheet and ActiveWorkbook?

+
ActiveSheet
refers to the worksheet currently displayed in Excel, while ActiveWorkbook
refers to the entire workbook currently open and active. Operations on ActiveSheet
will only affect the current sheet, whereas ActiveWorkbook
commands can impact the entire workbook.
How can I ensure my VBA code runs on a specific worksheet regardless of the active sheet?

+
You can reference a sheet by its name or index within the workbook. For example, Sheets(“Sheet1”).Activate
or Sheets(1).Activate
. This ensures your code operates on a specific sheet, regardless of the active sheet.
Is it possible to revert back to the original active worksheet after running a macro?

+
Yes, by storing a reference to the originally active sheet at the start of your macro. For example:
Dim originalSheet As Worksheet
Set originalSheet = ActiveSheet
’ Your code here
originalSheet.Activate