5 Ways to Copy Excel Worksheets Using VBA

Visual Basic for Applications (VBA) offers powerful tools to automate tasks in Microsoft Excel, significantly enhancing productivity. Among these tasks, copying worksheets is a common operation for many users. Whether you need to duplicate sheets for backup, distribute data, or modify templates, VBA can streamline this process. This blog will explore five distinct methods to copy Excel worksheets using VBA, detailing each approach with code snippets and practical applications.
Method 1: Using the Move or Copy Dialog Box

The simplest way to copy a worksheet is through Excel’s built-in interface:
- Right-click on the sheet tab you wish to copy.
- Select ‘Move or Copy.’
- In the dialog box, check the ‘Create a copy’ box.
- Choose where you want the copy to be placed.
This method can be automated using VBA with the following code:
Worksheets(“Sheet1”).Copy After:=Worksheets(“Sheet1”)
Method 2: Simple Copy with VBA
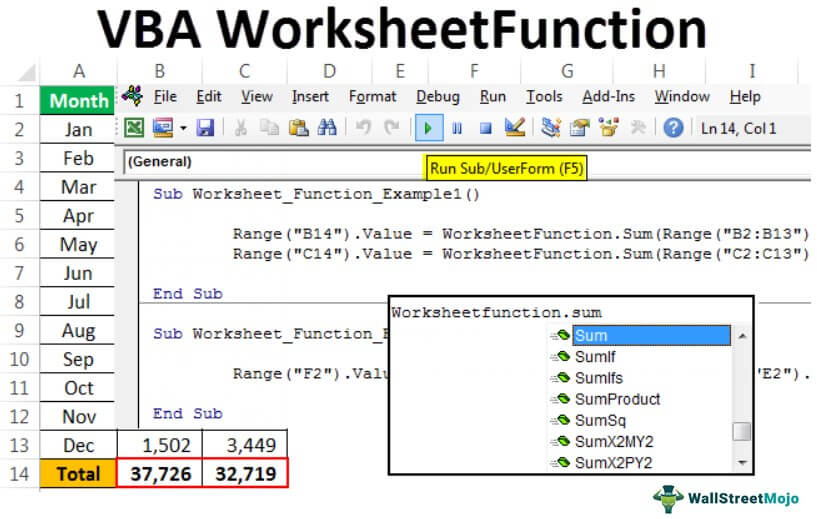
For a more direct approach, you can use VBA to copy a worksheet programmatically:
Sub CopySheet()
Sheets(“Sheet1”).Copy After:=Sheets(Sheets.Count)
End Sub
This script copies the active sheet (assuming “Sheet1”) to the end of the workbook. This is particularly useful for creating backups or when you need to duplicate sheets repeatedly.
Method 3: Copying to Another Workbook

Suppose you want to copy a worksheet to another workbook. Here’s how you can do it:
Sub CopyToAnotherWorkbook()
Dim wbSource As Workbook
Dim wbDest As Workbook
Set wbSource = ThisWorkbook
Set wbDest = Workbooks.Open("C:\Path\To\Your\Workbook.xlsx")
wbSource.Sheets("Sheet1").Copy After:=wbDest.Sheets(wbDest.Sheets.Count)
wbDest.Close True
End Sub
💡 Note: Ensure you replace the path with your destination workbook’s actual location.
Method 4: Copying with Formatting and Data

To copy a sheet including all formatting, charts, and formulas, you can use:
Sub FullCopySheet()
Sheets(“Sheet1”).Copy Before:=Sheets(1)
With Sheets(“Sheet1 (2)”)
.Cells.Copy
.Range(“A1”).PasteSpecial xlPasteValues
.Range(“A1”).PasteSpecial xlPasteFormats
.Range(“A1”).PasteSpecial xlPasteComments
Application.CutCopyMode = False
End With
End Sub
This method ensures that you capture all aspects of the original sheet, not just the visible cells.
Method 5: Conditional Copying

Sometimes, you might want to copy sheets based on certain conditions:
Sub ConditionalCopy()
For Each ws In ThisWorkbook.Worksheets
If ws.Range(“A1”).Value = “Copy” Then
ws.Copy After:=Worksheets(Worksheets.Count)
End If
Next ws
End Sub
Here, the code checks if cell A1 in each worksheet contains the text “Copy,” then copies those sheets to the end of the workbook. This is particularly useful in batch processing scenarios.
These five methods provide a comprehensive toolkit for copying worksheets in Excel using VBA. Whether you're looking to automate simple tasks or require complex conditional copying, there's a method suited for every need. Understanding these techniques not only boosts efficiency but also opens up new possibilities for data management and presentation.
Can VBA copy multiple sheets at once?

+
Yes, VBA can copy multiple sheets. You can either loop through sheets or directly reference multiple sheets in an array. For example:
Sub CopyMultipleSheets()
Sheets(Array(“Sheet1”, “Sheet2”, “Sheet3”)).Copy After:=Sheets(Sheets.Count)
End Sub
How can I ensure that only the values are copied, not the formulas?

+
You can use the xlPasteValues
option in the PasteSpecial
method to copy only the values:
Sub CopyValuesOnly()
Sheets(“Sheet1”).Range(“A1:Z100”).Copy
Sheets(“Sheet2”).Range(“A1”).PasteSpecial xlPasteValues
End Sub
What if I need to copy sheets with different names from different workbooks?

+
You would have to open each workbook, locate the sheet by its name, copy it, and then close the source workbook. Here’s a simplified example:
Sub CopyFromMultipleWorkbooks()
Dim wb1 As Workbook, wb2 As Workbook
Set wb1 = Workbooks.Open(“C:\Path\To\Workbook1.xlsx”)
Set wb2 = Workbooks.Open(“C:\Path\To\Workbook2.xlsx”)
wb1.Sheets("SheetA").Copy After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count)
wb2.Sheets("SheetB").Copy After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count)
wb1.Close False
wb2.Close False
End Sub