5 Types of Special Operators You Should Know

Understanding Special Operators in Programming

In programming, operators are symbols used to perform operations on variables and values. While most developers are familiar with basic arithmetic, comparison, and logical operators, special operators can significantly simplify code and improve readability. Here, we’ll explore five types of special operators that you should know, along with examples and explanations to help you master them.
1. Ternary Operator
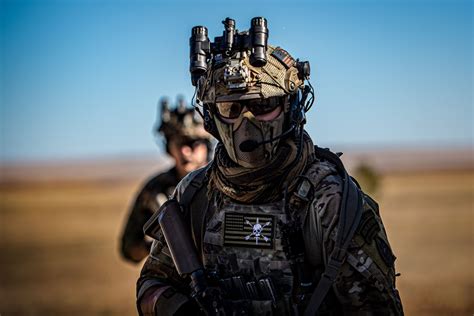
The ternary operator, also known as the conditional operator, is a shorthand way to write a simple if-else statement. It consists of three parts: the condition, the value if the condition is true, and the value if the condition is false.
Syntax: condition? value_if_true : value_if_false
Example:
let age = 25;
let status = age >= 18? 'adult' : 'minor';
console.log(status); // Output: "adult"
The ternary operator is useful when you need to assign a value to a variable based on a condition.
2. Spread Operator

The spread operator, denoted by three dots (...
), allows you to expand an array or an object into individual elements or properties. It’s commonly used to merge arrays, create new objects, or pass arguments to functions.
Syntax: ...array
or ...object
Example:
let numbers = [1, 2, 3];
let moreNumbers = [...numbers, 4, 5];
console.log(moreNumbers); // Output: [1, 2, 3, 4, 5]
The spread operator is useful when working with arrays and objects.
3. Rest Operator

The rest operator, also denoted by three dots (...
), is the opposite of the spread operator. It collects multiple elements or properties into an array or an object.
Syntax: function(...args)
or let {...obj } = object
Example:
function sum(...args) {
return args.reduce((a, b) => a + b, 0);
}
console.log(sum(1, 2, 3, 4, 5)); // Output: 15
The rest operator is useful when working with functions that accept a variable number of arguments.
4. Optional Chaining Operator

The optional chaining operator, denoted by ?.
, allows you to access properties or methods of an object without causing a null or undefined error.
Syntax: object?.property
or object?.method()
Example:
let user = { name: 'John', address: { street: '123 Main St' } };
console.log(user.address?.street); // Output: "123 Main St"
console.log(user.address?.city); // Output: undefined
The optional chaining operator is useful when working with nested objects or properties that may be null or undefined.
5. Nullish Coalescing Operator

The nullish coalescing operator, denoted by ??
, returns the first operand if it’s not null or undefined, and the second operand if it is.
Syntax: a?? b
Example:
let name = 'John';
let fullName = name?? 'Unknown';
console.log(fullName); // Output: "John"
let noName = null;
fullName = noName?? 'Unknown';
console.log(fullName); // Output: "Unknown"
The nullish coalescing operator is useful when working with variables that may be null or undefined.
In conclusion, mastering these special operators can simplify your code, improve readability, and reduce errors. By incorporating them into your programming workflow, you can write more efficient and effective code.
Mastering Special Operators: A Summary
- Ternary operator: shorthand for simple if-else statements
- Spread operator: expands arrays or objects into individual elements or properties
- Rest operator: collects multiple elements or properties into an array or object
- Optional chaining operator: accesses properties or methods without causing null or undefined errors
- Nullish coalescing operator: returns the first operand if it’s not null or undefined, and the second operand if it is
What is the purpose of the ternary operator?

+
The ternary operator is a shorthand way to write a simple if-else statement.
What is the difference between the spread and rest operators?

+
The spread operator expands an array or object into individual elements or properties, while the rest operator collects multiple elements or properties into an array or object.
When should I use the optional chaining operator?

+
Use the optional chaining operator when accessing properties or methods of an object that may be null or undefined.
Related Terms:
- Special Operations Command
- NATO Special Forces
- List of Special Forces
- Special Forces Operator salary
- Para SF
- U S Army Ranger