5 Ways to Rename Worksheets in VBA

Managing Excel spreadsheets efficiently can significantly streamline your workflow, especially when dealing with large datasets or complex workbooks. One common task in Excel VBA (Visual Basic for Applications) involves renaming worksheets. While this might seem simple, there are various scenarios where knowing different methods to rename worksheets can be particularly useful, enhancing both productivity and the user experience. Here are five insightful methods to rename worksheets using VBA:
Method 1: Using the Name Property
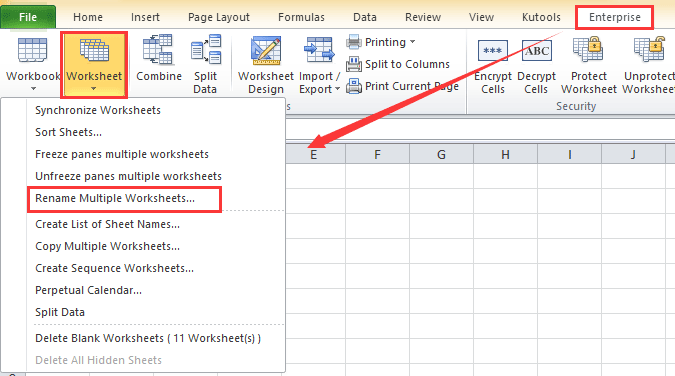
The most straightforward way to rename a worksheet is by directly setting the Name property of the Worksheet object. This method is ideal for basic renaming operations:
- Select the worksheet you want to rename.
- Change the name of the worksheet with a simple line of code:
Worksheets(“OldSheetName”).Name = “NewSheetName”
Here's an example:
Sub RenameSheet()
Worksheets("Sheet1").Name = "Sales Data"
End Sub
💡 Note: Remember that sheet names cannot exceed 31 characters and must be unique within the workbook.
Method 2: Error Handling for Duplicates

Handling potential conflicts with duplicate names is crucial. Here, we’ll use error handling to manage situations where the new sheet name might already exist:
Sub SafeRename()
On Error Resume Next
Worksheets(“Sheet1”).Name = “NewSheetName”
If Err.Number <> 0 Then
MsgBox “The worksheet name already exists or is not valid.”
Err.Clear
End If
On Error GoTo 0
End Sub
Method 3: Renaming Multiple Sheets Sequentially

Sometimes, you might need to rename multiple sheets at once, perhaps following a pattern. Here’s how you can do that:
- Loop through all the sheets in the workbook.
- Assign a new name to each sheet dynamically.
Sub RenameAllSheets()
Dim ws As Worksheet
Dim i As Integer
For i = 1 To ThisWorkbook.Sheets.Count
Set ws = ThisWorkbook.Sheets(i)
ws.Name = "Sheet" & i
Next i
End Sub
🔄 Note: Always ensure you have enough unique names for all sheets to avoid naming conflicts.
Method 4: Using InputBox for Interactive Renaming

For more user-friendly approaches, allowing users to input new names can be beneficial:
Sub InteractiveRename()
Dim NewName As String
NewName = InputBox(“Please enter a new name for the sheet:”)
If NewName = “” Then Exit Sub
Worksheets(“Sheet1”).Name = NewName
End Sub
Method 5: Renaming Based on Cell Values
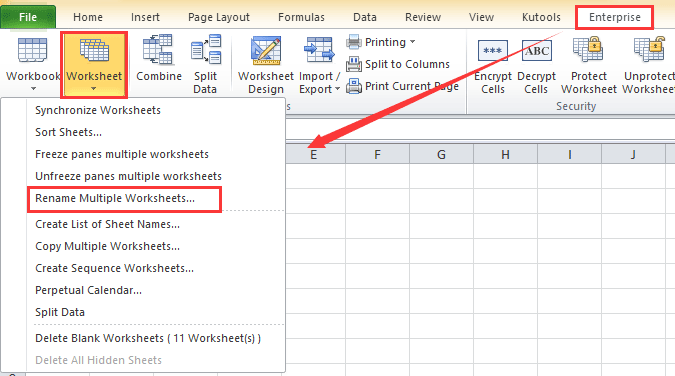
Automatically renaming sheets based on specific cell values within each sheet can save a lot of manual work:
- Access the cell’s value and set the sheet’s name accordingly.
Sub RenameFromCell()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If Not IsEmpty(ws.Range(“A1”).Value) Then
ws.Name = Left(ws.Range(“A1”).Value, 31) ‘ To ensure it fits within 31 characters
End If
Next ws
End Sub
📝 Note: Always validate the cell value to prevent naming errors or invalid sheet names.
The ability to rename worksheets dynamically using VBA can greatly enhance your Excel workflow, allowing for better organization and easier navigation through your workbooks. These methods provide a range of options from simple renames to more complex operations tailored to meet specific needs or conditions within your datasets.
The key points to remember include:
- Simple and Straightforward Renaming: Using the Name property for quick operations.
- Error Management: Safeguarding against naming conflicts or invalid names.
- Batch Renaming: Efficiently renaming multiple sheets at once.
- User Interaction: Incorporating user input for greater flexibility.
- Data-Driven Renaming: Utilizing cell content to automate renaming processes.
These techniques not only make Excel management easier but also demonstrate the power of VBA in automating mundane tasks, thereby allowing you to focus on more critical data analysis or reporting activities.
What happens if I try to rename a sheet to a name that already exists?

+
If you attempt to rename a sheet to a name already in use, VBA will raise an error. The error handling approach, as demonstrated, can manage this situation gracefully by prompting the user or by skipping the operation.
Can I rename sheets in a different workbook from the one where the VBA code is running?

+
Yes, you can rename sheets in another workbook by referencing that workbook. For example, Workbooks(“WorkbookName.xlsx”).Worksheets(“Sheet1”).Name = “NewSheetName”
.
How can I undo a VBA sheet rename operation?

+
VBA does not offer an undo feature for renaming. To undo, you would need to keep track of old names and manually or programmatically revert them. Alternatively, save the workbook before renaming to keep an old version.