5 Ways Remove Leading 0
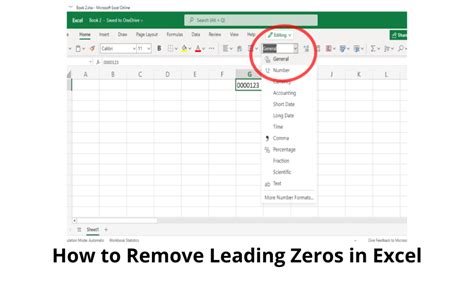
Introduction to Removing Leading Zeros

When working with numbers, especially in programming or data analysis, leading zeros can be a nuisance. They are often added to numbers to maintain a specific format or to ensure that numbers are correctly aligned in tables or spreadsheets. However, there are instances where these leading zeros need to be removed. This could be due to the requirement of a specific numerical format for input into a database, for further analysis, or simply for aesthetic reasons. In this article, we will explore 5 ways to remove leading zeros from numbers in various contexts.
Understanding Leading Zeros

Before diving into the methods of removing leading zeros, it’s essential to understand what they are. Leading zeros are zeros that appear at the beginning of a number. For example, in the number “0123”, the “0” at the start is a leading zero. These zeros do not affect the numerical value of the number but can cause issues in certain applications. Leading zeros can appear in integers, floats, or even in strings that represent numbers.
Method 1: Using Programming Languages

Many programming languages provide simple ways to remove leading zeros from numbers. For instance, in Python, you can convert a string representing a number with leading zeros to an integer, which automatically removes the leading zeros. Here’s an example:
number_str = "0123"
number_int = int(number_str)
print(number_int) # Outputs: 123
Similarly, in JavaScript, you can use the parseInt()
function or simply use the unary plus operator (+
) to achieve the same result:
let numberStr = "0123";
let numberInt = +numberStr;
console.log(numberInt); // Outputs: 123
Method 2: Using Spreadsheet Functions

In spreadsheet applications like Microsoft Excel or Google Sheets, you can remove leading zeros by changing the cell format to a number format that does not display leading zeros. Alternatively, you can use formulas to achieve this. For example, the
VALUE
function in Excel can convert a text string that represents a number (with leading zeros) into a number, effectively removing the leading zeros:
=VALUE(A1)
Assuming A1 contains the text “0123”, this formula will return the number 123.
Method 3: Using Text Editors

Many text editors, including Notepad++ and Sublime Text, offer regular expression (regex) find and replace functionality. You can use regex to find and remove leading zeros from numbers in a text file. The regex pattern
^0+
can be used to match one or more leading zeros at the start of a line. By replacing matches with an empty string, you effectively remove the leading zeros.
Method 4: Using Command Line Tools

For those comfortable with the command line, tools like
sed
(stream editor) can be very powerful for removing leading zeros from numbers in text files. The command:
sed 's/^0*//'
will remove leading zeros from the start of each line in a file.
Method 5: Manual Removal
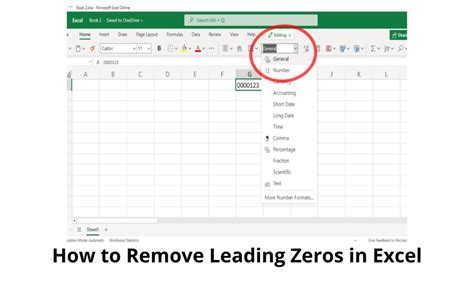
Finally, for small datasets or one-off instances, manually removing leading zeros might be the quickest solution. This involves simply deleting the leading zeros from the number. While not efficient for large datasets, it’s a straightforward approach for occasional use.
📝 Note: When removing leading zeros, ensure that the resulting number is in the correct format for its intended use, especially if the number is to be used in a specific context like a database or further numerical analysis.
To summarize, removing leading zeros can be accomplished through various methods, each suited to different contexts and user preferences. Whether through programming, spreadsheet functions, text editors, command line tools, or manual removal, there’s a way to efficiently remove leading zeros to meet your needs.
Why are leading zeros a problem in some applications?

+
Leading zeros can be a problem because they might not be recognized as part of a numerical value in certain contexts, such as in programming or when importing data into a database. They can also cause formatting issues in tables and spreadsheets.
Can leading zeros be removed from all types of numbers?

+
Leading zeros can be removed from integers and floats, but the method might vary depending on the context (e.g., programming language, spreadsheet). However, numbers that are meant to have leading zeros, like phone numbers or specific identifiers, should not have their leading zeros removed as it could alter their meaning or functionality.
What is the most efficient way to remove leading zeros from a large dataset?

+
The most efficient way often involves using programming languages or command line tools that can process the data in bulk. This approach minimizes manual effort and can handle large datasets quickly.