5 Ways to Manage VBA Worksheet Names Effectively

Effective management of VBA worksheet names in Excel can streamline your work, making it easier to organize, navigate, and maintain large data sets. Here are five proven techniques to manage your worksheet names efficiently:
1. Automate Worksheet Naming


Automating the naming process reduces errors and ensures consistency. Here’s how:
- Create a Naming Template: Define a standard format for your worksheet names.
- Use VBA Code: Develop a macro that applies your naming template. For example:
Sub AutoNameSheets() Dim ws As Worksheet Dim counter As Integer counter = 1 For Each ws In ThisWorkbook.Worksheets ws.Name = "Sheet_" & counter counter = counter + 1 Next ws End Sub
</li>
💡 Note: Keep in mind that Excel has limitations on sheet names, such as a maximum of 31 characters, no duplicate names, and restricted characters like “:”,“*”,“?”,“/”,“[”, or “]”.
2. Batch Rename Worksheets


When dealing with multiple worksheets, renaming them in one go saves time. Here’s how to do it:
- Create a UserForm: Develop a userform with fields for current names and new names.
- Implement a Macro: Use the following code to execute batch renaming:
Sub BatchRename() Dim ws As Worksheet Dim newNames() As String newNames = Array("Report", "Data", "Sales", "Marketing") For Each ws In ThisWorkbook.Worksheets If ws.Index <= UBound(newNames) + 1 Then ws.Name = newNames(ws.Index - 1) End If Next ws End Sub
</li>
3. Dynamic Naming


Dynamically changing worksheet names based on certain criteria or data can be particularly useful. Here’s how:
- Determine the Trigger: Identify what event or data change will trigger the name change.
- Write VBA Code: Use events or conditions to rename sheets on the fly:
Private Sub Worksheet_Change(ByVal Target As Range) If Not Intersect(Target, Me.Range("A1")) Is Nothing Then If Me.Range("A1").Value <> "" Then Me.Name = Me.Range("A1").Value End If End If End Sub
</li>
💡 Note: The above code must be placed in the Worksheet module and not in a standard module.
4. Error Handling and Validation


Avoiding errors when renaming sheets is crucial:
- Implement Error Checking: Use error handling to manage invalid names or duplicates.
- Create a Validation Function: Ensure names meet Excel’s criteria:
Function isValidSheetName(name As String) As Boolean isValidSheetName = Len(name) > 0 And _ Len(name) <= 31 And _ Not WorksheetExists(name, ThisWorkbook) And _ Not InStr(1, name, ":") > 0 And _ Not InStr(1, name, "*") > 0 End Function Function WorksheetExists(sName As String, oWbk As Workbook) As Boolean On Error Resume Next WorksheetExists = Not oWbk.Worksheets(sName) Is Nothing End Function
</li>
5. Maintain History


Keeping a log of name changes can be useful for tracking changes and auditing:
- Set Up a Log Sheet: Create a separate worksheet for logging changes.
- Automate Logging: Use the following VBA code to log changes:
Sub LogSheetRename(newName As String, oldName As String) Dim logSheet As Worksheet Set logSheet = ThisWorkbook.Sheets("SheetRenameLog") Dim lastRow As Long lastRow = logSheet.Cells(logSheet.Rows.Count, 1).End(xlUp).Row + 1 logSheet.Cells(lastRow, 1).Value = "Sheet " & oldName & " renamed to " & newName logSheet.Cells(lastRow, 2).Value = Now() End Sub
</li>
💡 Note: A well-organized workbook with consistent naming makes maintenance and collaboration more efficient.
To wrap up, mastering the management of worksheet names with VBA in Excel involves automation, validation, and tracking changes. By following these five methods, you can ensure your workbooks remain organized, allowing for faster access, reduced errors, and enhanced productivity. These techniques will not only streamline your own work but also make it easier for others to navigate through your Excel files.
Can you rename worksheets dynamically based on data within the sheet?
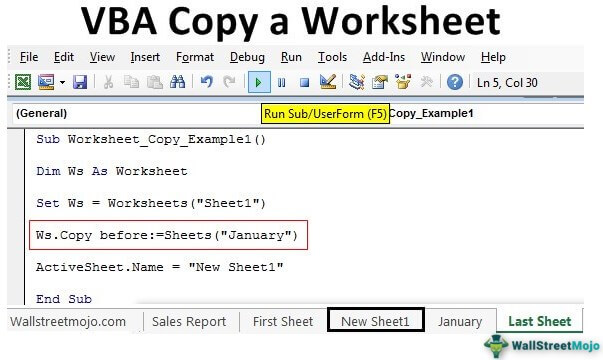
+
Yes, you can set up VBA events or macros to automatically rename a worksheet based on the data entered or changed within specific cells.
What are the limitations when renaming worksheets in Excel?

+
Excel limits sheet names to 31 characters, prohibits duplicates, and disallows certain characters like colons, asterisks, question marks, forward slashes, square brackets, and periods in the first or last position.
How can I prevent errors when renaming multiple worksheets?

+
Implement error handling and validation checks within your VBA code to manage invalid names or duplicates, ensuring smooth batch renaming processes.