5 Essential VBA Worksheet Cells Hacks

Utilizing the Power of VBA

Visual Basic for Applications (VBA) is a powerful tool that enables automation, customization, and manipulation of Microsoft Excel. For professionals and enthusiasts alike, understanding how to leverage VBA, particularly when working with worksheet cells, can vastly enhance productivity and functionality. This post explores five essential hacks for working with VBA worksheet cells, transforming complex tasks into simplified solutions.
1. Simplifying Range Operations with Named Ranges

Excel allows you to define named ranges, which can make your VBA code more readable and maintainable. Instead of using cumbersome cell addresses, you can refer to ranges by meaningful names.
Sub UseNamedRange()
' Define a named range
ThisWorkbook.Names.Add Name:="EmployeeData", RefersTo:="=Sheet1!$A$2:$D$50"
' Now you can work with the named range easily
Range("EmployeeData").Value = Array("Name", "Age", "Department", "Salary")
End Sub
💡 Note: Remember that named ranges are workbook-wide, so ensure you are in the correct worksheet before using them.
2. Autofit Columns and Rows for Better Readability

When working with data, ensuring that the columns and rows adjust to fit their contents automatically can enhance readability. Here’s how you can automate this process using VBA:
Sub AutofitWorksheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
' Autofit all columns
ws.Cells.EntireColumn.AutoFit
' Autofit all rows
ws.Cells.EntireRow.AutoFit
End Sub
3. Dynamically Copy and Paste Data

Copying and pasting data within Excel can be a tedious task if done manually, especially with large datasets. VBA provides a seamless way to automate this:
Sub CopyAndPaste()
Dim sourceSheet As Worksheet, targetSheet As Worksheet
Set sourceSheet = ThisWorkbook.Sheets("SourceData")
Set targetSheet = ThisWorkbook.Sheets("TargetData")
' Clear previous data from the target sheet
targetSheet.Cells.Clear
' Copy the entire used range from the source sheet
sourceSheet.UsedRange.Copy targetSheet.Range("A1")
End Sub
💡 Note: The UsedRange method includes all cells that have ever been used, even if they are currently blank, which might affect performance for large spreadsheets.
4. Filtering Data with Advanced Criteria

Advanced filtering allows you to extract specific data from a larger dataset based on complex criteria, which can be particularly useful for analysis:
Sub AdvancedFilter()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("EmployeeData")
' Assuming A1:D50 contains data and A51 contains criteria
ws.Range("A1:D50").AdvancedFilter Action:=xlFilterInPlace, CriteriaRange:=ws.Range("A51:A52"), Unique:=False
End Sub
5. Inserting Hyperlinks Dynamically

Hyperlinks in Excel can guide users to different locations, either within the workbook, another workbook, or on the web. Here’s how to dynamically insert hyperlinks:
Sub AddHyperlinks()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Links")
Dim cell As Range
' Loop through cells in column A where hyperlink information is stored
For Each cell In ws.Range("A2:A" & ws.Cells(ws.Rows.Count, 1).End(xlUp).Row)
If cell.Value <> "" Then
' Add a hyperlink to the cell
cell.Hyperlinks.Add Anchor:=cell, Address:="https://www.example.com", TextToDisplay:=cell.Value
End If
Next cell
End Sub
Understanding these VBA hacks opens a world of efficiency and automation in Excel. Whether you're dealing with large datasets or repetitive tasks, these techniques can significantly cut down the time spent on data manipulation, allowing for more focus on analysis and decision-making.
Remember, the key to mastering VBA is practice and understanding Excel's object model. Start with these hacks, experiment with variations, and you'll soon find yourself manipulating Excel data with ease and precision.
What is VBA?
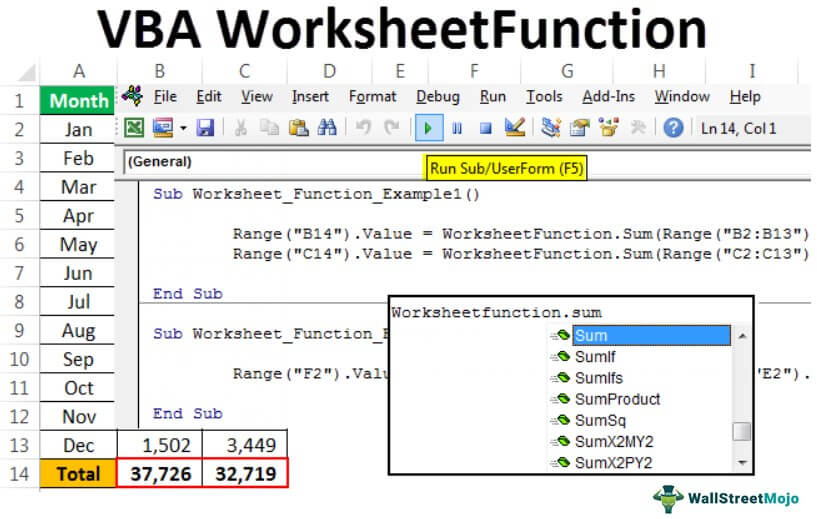
+
VBA stands for Visual Basic for Applications, a programming language developed by Microsoft to automate tasks in Office applications.
Can I run VBA macros on any version of Excel?

+
Yes, VBA macros are supported across most versions of Microsoft Excel, although compatibility might differ between very old or new versions.
How do I enable macros in Excel?
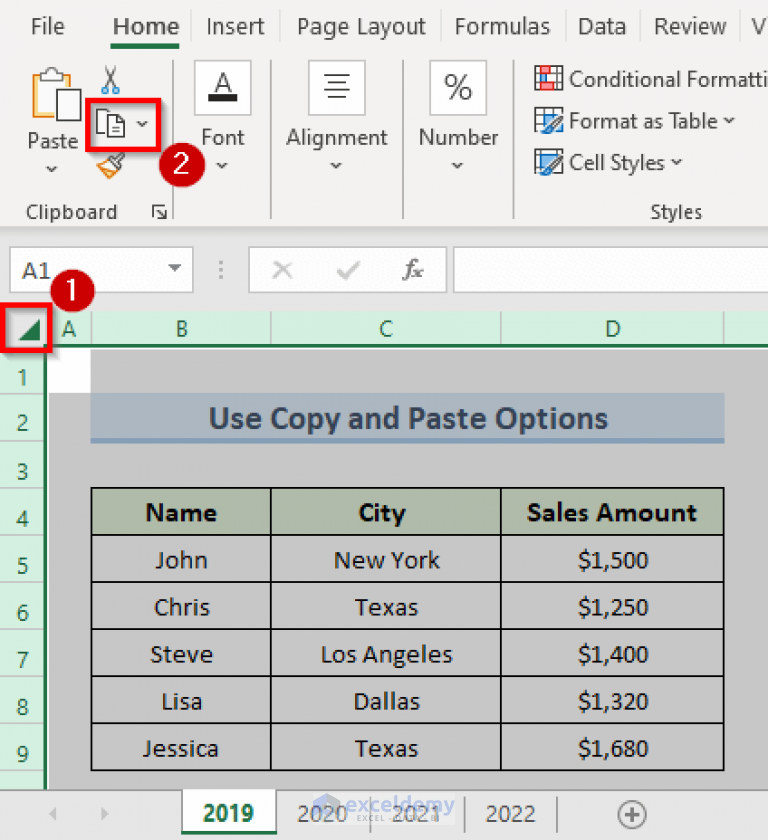
+
Go to the File tab, select Options, then Trust Center, and click on Trust Center Settings. Enable macros under Macro Settings.