Master Variables Easily with This Solving Worksheet

Understanding and mastering variables is one of the foundational skills in any programming language. Whether you are a beginner or an experienced developer, the concept of variables remains crucial because it helps you store, retrieve, and manipulate data efficiently. This blog post will guide you through a comprehensive worksheet designed to make variables as straightforward and intuitive as possible. By the end, you'll be able to confidently handle variables in your code with ease and precision.
Why Variables Matter in Programming
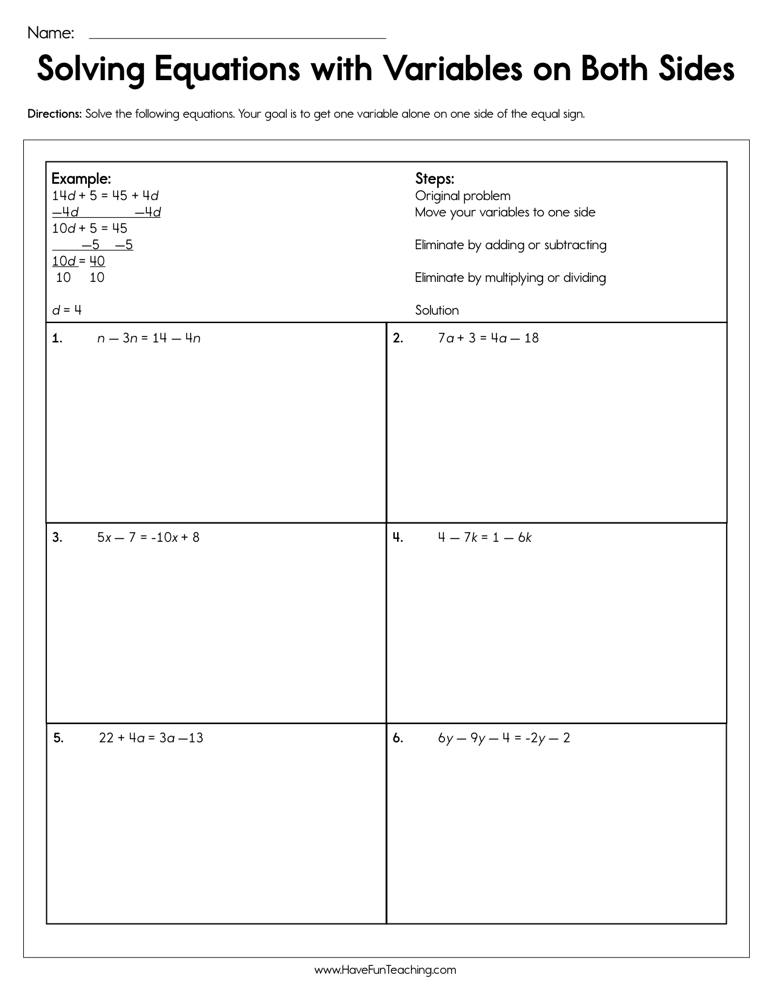
Variables are the backbone of any program, acting as containers for storing data values. They enable the programmer to work with dynamic data, respond to user inputs, and manage complex algorithms by simplifying data manipulation. Here are some key reasons why variables are indispensable:
- Memory Efficiency: Variables help in reusing memory by not hardcoding values, which leads to efficient memory use.
- Code Readability and Maintainability: Descriptive variable names make the code easier to understand and maintain.
- Dynamic Programming: Variables facilitate the execution of programs that respond to different inputs or conditions dynamically.
- Debugging and Testing: By altering the values of variables, developers can test different scenarios and debug code effectively.
Getting Started with Variables

Let’s dive into a practical worksheet that will help you master variables. This section assumes basic knowledge of programming terminology and will use Python for examples, but the concepts apply universally.
Step 1: Declare and Initialize Variables

First, we need to declare and initialize variables. This means assigning a value to a variable for the first time. Here are some examples:
# Integer variable
age = 25
# String variable
name = "Alice"
# Float variable
height = 5.7
📝 Note: Ensure variable names are descriptive but concise to improve code readability. In most programming languages, variable names cannot contain spaces or special characters, except for underscores (_).
Step 2: Assigning New Values to Variables

After declaring variables, you can reassign their values. This flexibility is one of the key features of variables:
# Reassign integer
age = age + 1 # Alice is now one year older
# Reassign string
name = "Bob"
# Reassign float
height += 0.2
Step 3: Using Variables in Operations

Variables are not just for storage; they are pivotal in performing operations. Here’s how you might use them:
# Arithmetic Operations
total_height = height * 2 # Assume Bob's height has doubled for some reason
# String Concatenation
message = f"Hello, {name}! You are {age} years old."
# Conditionals
if age >= 18:
print("You are an adult.")
Type of Variable | Example |
---|---|
Integer | age = 25 |
String | name = "Alice" |
Float | height = 5.7 |
Boolean | is_student = True |

Practical Exercises

Here are a series of exercises to solidify your understanding:
Exercise 1: Summing Numbers
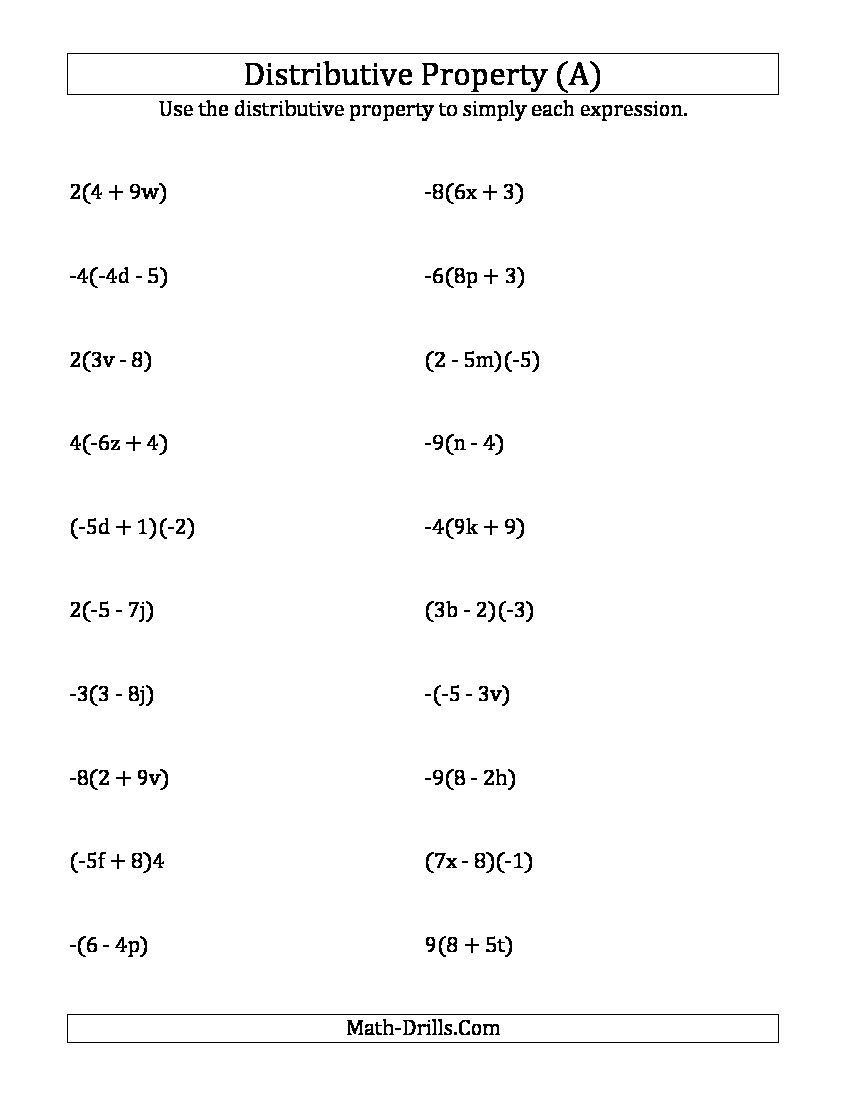
Create two integer variables, assign them values, and then add their values to a third variable.
num1 = 10
num2 = 20
sum_numbers = num1 + num2
Exercise 2: String Manipulation

Create a string variable and use slicing or concatenation to modify it.
name = "Elise"
new_name = name[0] + "el" # 'Eel'
📝 Note: String manipulation is often one of the trickiest parts of variable usage. Practice different techniques like slicing, indexing, or using string methods to improve your skills.
Exercise 3: Temperature Conversion

Write a program that converts Celsius to Fahrenheit using variables.
celsius = 25
fahrenheit = (celsius * 9/5) + 32
print(f"{celsius}°C is {fahrenheit}°F")
Advanced Techniques

Now that you’ve got the basics down, let’s look at some advanced techniques for working with variables:
Variable Scopes

Understanding variable scopes is crucial:
- Local Variables: Variables declared within a function. They are accessible only within that function.
- Global Variables: Variables declared outside of a function that can be accessed by any part of the program.
- Static Variables: In some languages, variables that retain their value between function calls.
# Global Variable
greeting = "Hello"
def greet(name):
# Local Variable
welcome = "Welcome"
print(f"{greeting}, {name}! {welcome} to the program!")
Dynamic Typing
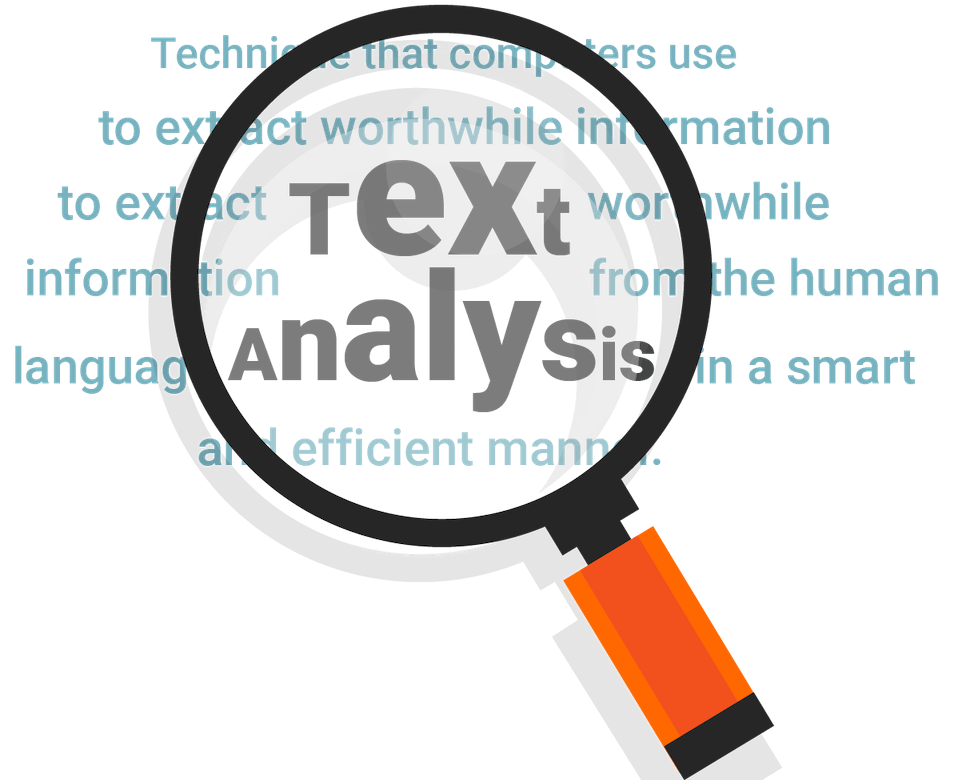
Python uses dynamic typing, where the variable’s type is determined by the value it holds:
x = 5 # x is now an integer
x = "Hello" # x is now a string
This flexibility can be powerful but requires careful coding to avoid type-related errors.
In summary, variables are the lifeblood of programming, allowing for dynamic data manipulation and interaction. This worksheet has covered basic to advanced concepts, ensuring you’re well-equipped to handle variables confidently. From initializing and assigning values to understanding scopes and dynamic typing, you’ve embarked on a journey of learning and practice. Keep coding and experimenting with variables to deepen your understanding and harness the full potential of programming.
Why is variable declaration important?

+
Variable declaration is crucial because it allocates memory space for storing data, ensuring efficient memory usage, readability, and maintainability of code. It also helps in type checking and error prevention in statically typed languages.
Can I change the type of a variable after initialization?
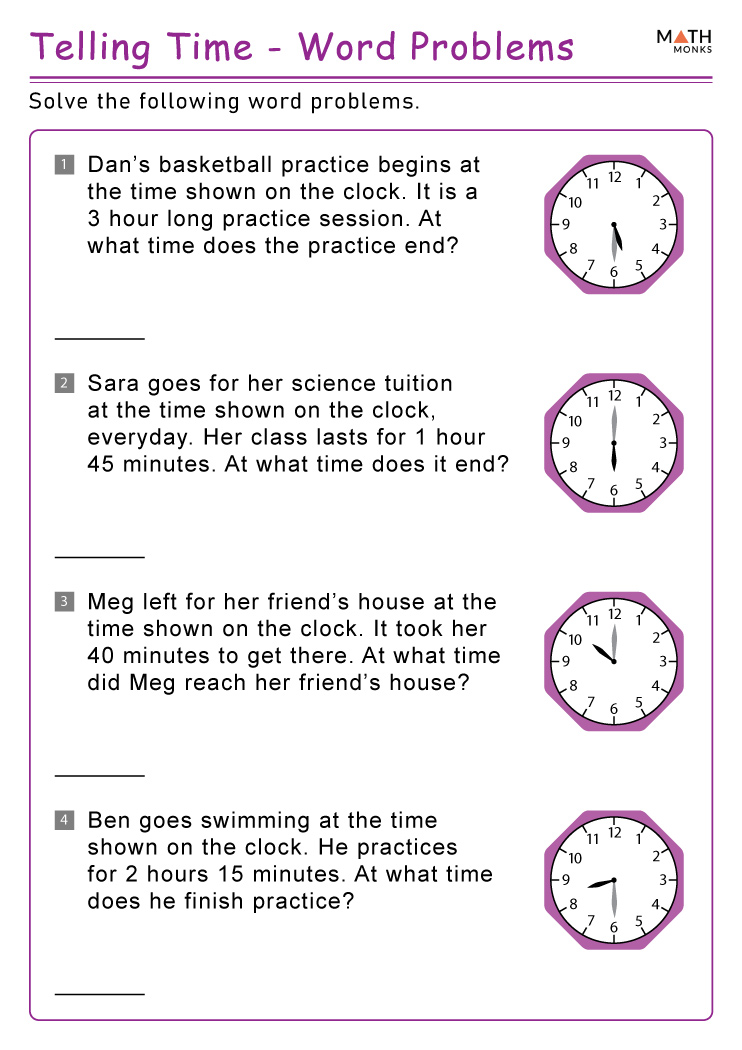
+
Yes, in dynamically typed languages like Python, JavaScript, or Ruby, you can reassign a variable to hold a value of a different type. However, be cautious as this can lead to type-related errors if not managed properly.
What’s the difference between a variable and a constant?
+A variable is a container for data that can change its value during the execution of the program. A constant, however, holds a value that should not be modified once it is set. Constants are typically used for values that should remain constant throughout the program’s lifecycle, like configuration settings or mathematical constants.