5 Simple VBA Tricks to Rename Worksheets Quickly

Managing Excel workbooks can sometimes be a cumbersome task, especially when dealing with numerous worksheets. However, with Visual Basic for Applications (VBA), you can automate repetitive tasks like renaming worksheets quickly and efficiently. In this detailed guide, we will explore five simple VBA tricks that will help you rename worksheets in Excel without breaking a sweat. Whether you're a beginner or an intermediate user looking to enhance your VBA skills, these tricks will provide you with a solid foundation to streamline your workflow.
1. Renaming a Single Worksheet with VBA
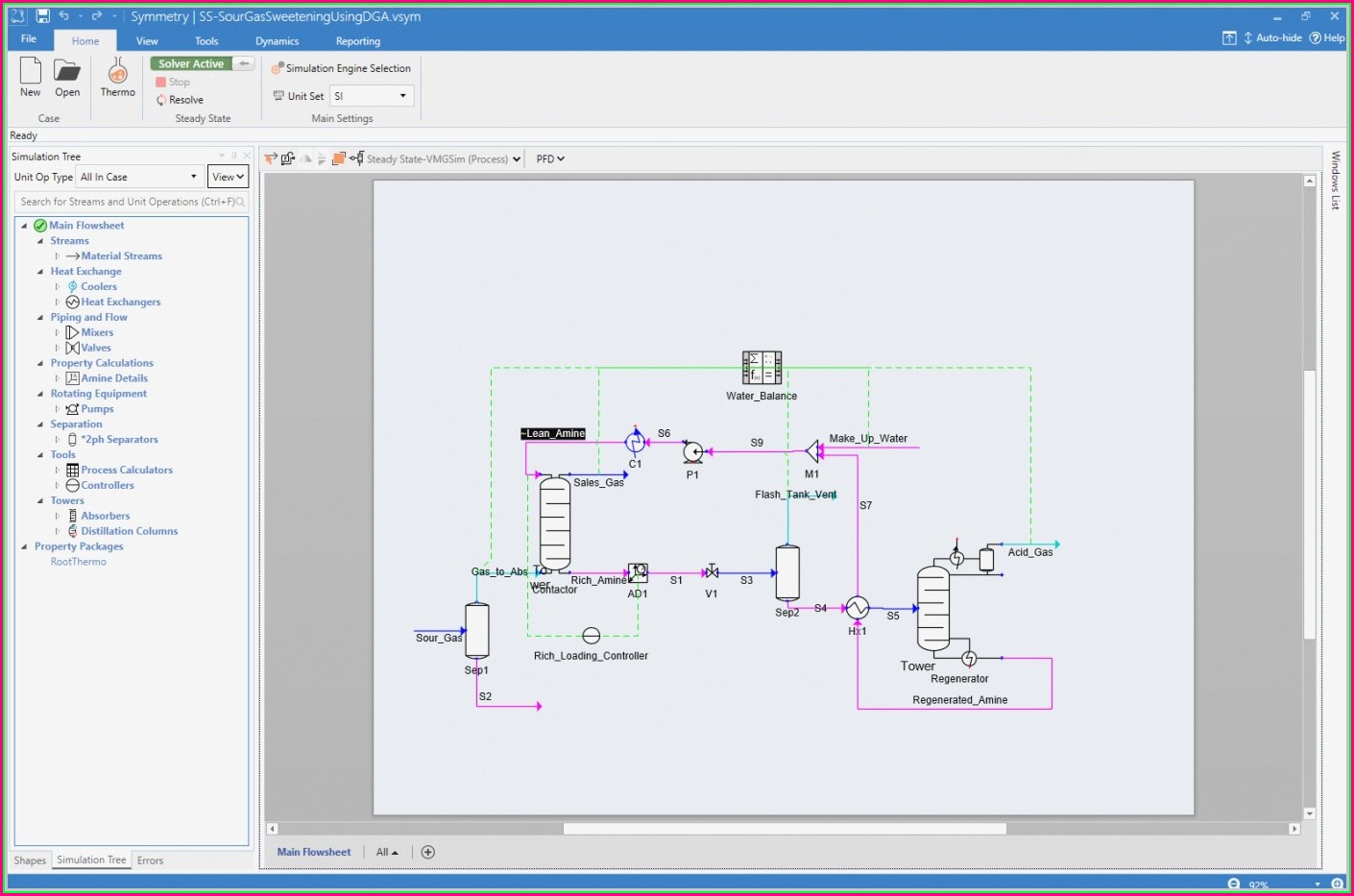
The most basic operation in VBA when it comes to renaming sheets involves changing the name of one specific worksheet. Here's how you can do it:
- Press ALT + F11 to open the VBA editor in Excel.
- Insert a new module by right-clicking on any of the objects in the "Project Explorer" and choosing "Insert" > "Module".
- Type or paste the following VBA code:
Sub RenameSingleSheet()
ActiveSheet.Name = "New Sheet Name"
End Sub
đź’ˇ Note: Make sure the sheet you want to rename is active when you run the macro.
2. Renaming Multiple Sheets Sequentially

If you have several sheets that need to be renamed sequentially, VBA can handle this effortlessly. Here’s a script to rename multiple sheets in order:
- Follow the steps to open the VBA editor and insert a module.
- Use the following VBA code:
Sub RenameSheetsInSequence()
Dim ws As Worksheet
Dim i As Integer
i = 1
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> "Sheet1" Then
ws.Name = "Sheet" & i
i = i + 1
End If
Next ws
End Sub
This macro will rename all sheets in the workbook except "Sheet1" with the prefix "Sheet" followed by a sequential number. Remember to replace "Sheet1" with the name of the sheet you wish to keep unchanged or remove the condition if you want to rename all sheets.
3. Renaming Sheets Based on Cell Content

Often, you might want to name sheets dynamically based on the data within them. Here's how to rename sheets using data from a specific cell:
- Open the VBA editor and insert a module.
- Enter the following code:
Sub RenameSheetByCellContent()
Dim ws As Worksheet
For Each ws In ActiveWorkbook.Worksheets
If ws.Range("A1").Value <> "" Then
ws.Name = Left(ws.Range("A1").Value, 31)
End If
Next ws
End Sub
This macro checks the value in cell A1 of each worksheet. If there is a value, it will rename the sheet with the contents of A1, truncated to 31 characters (the maximum length for a sheet name). Adjust the cell reference (A1) to fit your data placement needs.
4. Using a Custom Function to Validate Sheet Names

Before renaming sheets, it's wise to check if the new name is valid. Excel has rules for what characters can be used in sheet names:
- Open the VBA editor, insert a module, and add this function:
Function IsValidSheetName(strName As String) As Boolean
IsValidSheetName = True
If Len(strName) > 31 Or Len(strName) = 0 Then
IsValidSheetName = False
ElseIf InStr(strName, ":") > 0 Or _
InStr(strName, "\") > 0 Or _
InStr(strName, "/") > 0 Or _
InStr(strName, "?") > 0 Or _
InStr(strName, "*") > 0 Or _
InStr(strName, "[") > 0 Or _
InStr(strName, "]") > 0 Then
IsValidSheetName = False
End If
End Function
You can then use this function in any macro that involves renaming sheets to ensure the name adheres to Excel's naming conventions. Here’s an example:
Sub RenameWithValidation()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If IsValidSheetName("New Sheet Name") Then
ws.Name = "New Sheet Name"
Else
MsgBox "Invalid Sheet Name"
End If
Next ws
End Sub
By integrating this validation, you avoid errors when renaming sheets due to invalid characters or name length issues.
5. Batch Renaming Sheets with a Dialog Box

For a user-friendly experience, you can create a macro that prompts for new sheet names through a dialog box:
- In the VBA editor, insert a UserForm by choosing "Insert" > "UserForm".
- Add a TextBox and a CommandButton to the form, and set their properties as needed.
- Create this code behind the CommandButton_Click event:
Private Sub CommandButton1_Click()
Dim ws As Worksheet
Dim i As Integer
i = 0
For Each ws In ThisWorkbook.Worksheets
If i < TextBox1.Value Then
ws.Name = "Sheet" & i
i = i + 1
Else
Exit For
End If
Next ws
MsgBox "Sheets Renamed!"
Me.Hide
End Sub
This script allows users to enter the number of sheets they want to rename, up to the total number of sheets in the workbook. After entering the number, clicking the button will rename the sheets accordingly.
In wrapping up, these VBA tricks offer a versatile toolkit for anyone looking to manage Excel worksheets more effectively. Each trick adds a layer of automation, reducing manual efforts and enhancing productivity. Whether it’s renaming a single sheet, a batch of sheets, or dealing with dynamic naming conventions, VBA provides an answer. By incorporating these techniques into your daily tasks, you can work smarter, not harder, making Excel an even more powerful tool for data management.
Can I rename Excel sheets without using VBA?

+
Yes, you can rename sheets manually by right-clicking the sheet tab and selecting “Rename”. However, VBA allows you to automate and customize this process.
What are the limitations of renaming sheets with VBA?

+
The limitations include: - Sheet names must be unique in a workbook. - Sheet names cannot exceed 31 characters. - Certain characters like “:” or “/” are not allowed in sheet names.
Is it possible to undo sheet renaming with VBA?
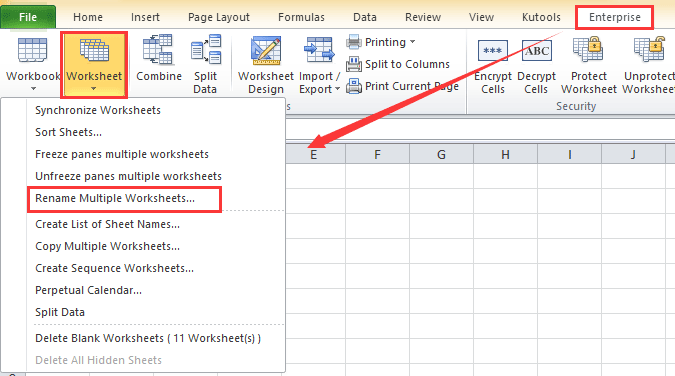
+
While there isn’t a direct “undo” function for VBA, you can create a subroutine that stores original names before renaming and then reverts them if needed.
Related Terms:
- Copy and rename worksheet VBA
- Delete sheet VBA
- VBA add sheet with name
- Rename sheet with date VBA