5 Essential Tips for Using Range.worksheet in VBA

The Microsoft Excel object model provides VBA developers with a rich set of tools to manipulate and automate Excel spreadsheets. One of the most versatile and powerful tools at your disposal is the Range.Worksheet property, which allows you to interact with worksheet data efficiently. In this extensive guide, we will explore five essential tips to master the use of Range.Worksheet in VBA, ensuring that you can handle your Excel automation tasks with expertise and precision.
1. Understanding the Basics

Before diving into the advanced tips, it’s crucial to understand the basics of Range.Worksheet.
- Definition: The Worksheet property of a Range object returns the worksheet that contains the range.
- Usage: This property can be used to reference the parent worksheet directly from any range within it.
Here is a simple example:
Dim ws As Worksheet
Set ws = Range("A1").Worksheet
MsgBox ws.Name
This code snippet demonstrates retrieving the name of the worksheet in which cell A1 is located.
Example Applications:

- Verifying the worksheet where data operations will be performed.
- Using the parent sheet for performing operations like copying or formatting.
2. Efficient Data Entry with Range.Worksheet
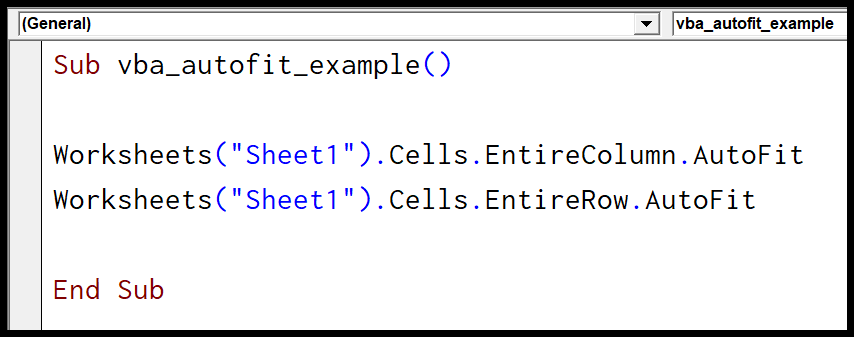
When automating data entry, using Range.Worksheet can streamline your process:
- Create Worksheets: If you need to dynamically create worksheets, you can use Range.Worksheet after creation to interact with the new sheet.
- Data Validation: Ensure that data input aligns with the correct sheet to avoid errors or misplaced entries.
Here's how you can use it for efficient data entry:
With ThisWorkbook.Sheets.Add
.Name = "New_Data"
Range("A1").Worksheet.Name = "New_Data"
Range("A1:B10").Value = Array(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
End With
This code creates a new worksheet, names it, and then uses Range.Worksheet to populate cells with an array of numbers.
⚠️ Note: Ensure you have sufficient system resources when adding new sheets programmatically as it could lead to performance issues if done excessively.
3. Automation of Worksheet Navigation

Automating navigation within a workbook can significantly enhance productivity. Here’s how Range.Worksheet can be utilized:
- Reference Other Sheets: You can use a range from one sheet to access another for data operations.
- Loop Through Sheets: Use a loop to move through worksheets and perform tasks:
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
Debug.Print ws.Name
Next ws
This snippet shows how to loop through all worksheets and print their names, allowing you to navigate through the workbook programmatically.
4. Data Manipulation

When manipulating data within Excel, understanding how to use Range.Worksheet effectively can save time and reduce errors:
- Conditional Formatting: Apply formatting rules to specific worksheets.
- Data Filtering: Filter data on different sheets based on your criteria.
- Data Copy/Paste: Transfer data between worksheets efficiently.
Here’s an example to demonstrate these capabilities:
With Range("A1:B10").Worksheet
.Range("A1:B10").FormatConditions.Add Type:=xlCellValue, Operator:=xlGreater, Formula1:="=10"
.Range("A1:B10").FormatConditions(1).Interior.Color = vbYellow
End With
This code applies a conditional formatting rule to highlight cells greater than 10 in yellow.
🧑💻 Note: Always be cautious when automating formatting or data manipulation on large datasets to prevent crashes or timeout errors.
5. Error Handling and Safe Execution

Working with Excel involves dealing with various potential errors. Here are some techniques for using Range.Worksheet safely:
- Check for Existence: Before manipulating a range or worksheet, check if it exists.
- Error Trapping: Implement error handling to catch and manage unexpected errors gracefully.
- Undo/Cleanup Actions: Provide mechanisms to undo changes or clean up data if something goes wrong.
Here’s how you can integrate error handling:
On Error Resume Next
Dim ws As Worksheet
Set ws = Range("A1").Worksheet
If ws Is Nothing Then
MsgBox "Worksheet not found."
Else
MsgBox "Worksheet Name: " & ws.Name
End If
On Error GoTo 0
This code snippet showcases error trapping to check for a worksheet's existence before attempting any operations on it.
By following these tips, you will not only enhance your Excel VBA programming skills but also build more robust, reliable, and scalable Excel applications. Whether you are managing data entry, automating report generation, or just organizing spreadsheets, leveraging the Range.Worksheet property can make your tasks much more manageable and efficient. In summary, mastering Range.Worksheet in VBA: - Helps in understanding and controlling data flow within and across sheets. - Enhances data entry and manipulation accuracy. - Facilitates automation of workbook navigation and data processing. - Ensures safer execution with built-in error handling mechanisms.
Keep these tips in mind as you develop your Excel VBA solutions, and you'll be well-equipped to handle most challenges Excel can throw at you. With a little practice, you’ll be able to manipulate Excel with finesse, making your automation tasks smoother and more professional.
What is the purpose of using Range.Worksheet in VBA?

+
The Range.Worksheet property in VBA is used to get a reference to the worksheet where a particular range exists. This is crucial for operations that require interaction with the parent worksheet of a cell or range, like formatting, data validation, or navigating through different sheets within a workbook.
Can Range.Worksheet be used to manipulate data in another worksheet?

+
Yes, Range.Worksheet can indirectly help with manipulating data in other worksheets. By accessing the parent sheet of one range, you can then reference other sheets or ranges for data manipulation or copying operations.
How can I check if a specific worksheet exists using VBA?

+
To check if a specific worksheet exists, you can loop through the Worksheets collection or use error handling with Worksheet or Range.Worksheet references:
On Error Resume Next Dim ws As Worksheet Set ws = ThisWorkbook.Sheets(“SheetName”) If ws Is Nothing Then MsgBox “Worksheet not found.”