5 Ways to Apply VBA Code to All Sheets

Have you ever found yourself in a situation where you need to apply the same VBA code to all worksheets in an Excel workbook? Whether you're standardizing formatting, performing batch calculations, or implementing complex logic across multiple sheets, using Visual Basic for Applications (VBA) can be a lifesaver. Let's dive into five methods to ensure your VBA code touches every sheet in your workbook effectively.
Method 1: Loop Through All Sheets

The simplest and most common approach to applying VBA to all sheets involves using a for-each loop to iterate through each sheet in the workbook. Here’s how you can do it:
Sub LoopThroughSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Cells.ClearFormats
ws.Cells.RowHeight = 20
ws.Cells.ColumnWidth = 15
Next ws
End Sub
This VBA script clears formats and sets a uniform row height and column width for every sheet in the active workbook.
🗝️ Note: Make sure to include error handling to manage sheets that might not support all changes.
Method 2: Apply to Active Sheets Only

Sometimes, you don’t need to apply your VBA code to all sheets, just the ones currently open or visible. Here’s a script for that scenario:
Sub LoopThroughActiveSheets()
Dim ws As Worksheet
For Each ws In ActiveWindow.SelectedSheets
ws.Range("A1:A100").Value = "New Data"
Next ws
End Sub
👁️ Note: The script will only affect the sheets you've selected in the current window.
Method 3: Use an Array of Sheet Names
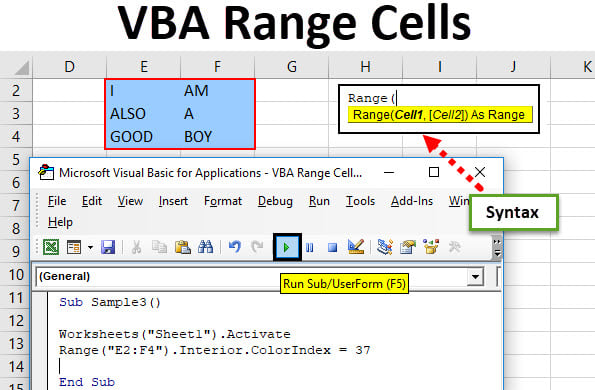
If you’re interested in targeting specific sheets by name, using an array can be an excellent method:
Sub ApplyToSpecificSheets()
Dim sheetNames As Variant
sheetNames = Array("Sheet1", "Sheet3", "DataAnalysis")
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If Not IsError(Application.Match(ws.Name, sheetNames, 0)) Then
ws.Cells.Interior.Color = RGB(255, 255, 0)
End If
Next ws
End Sub
This approach allows for flexibility by specifying which sheets to modify.
Method 4: Use Workbook-Level Events

For continuous application of VBA to new or existing sheets, leveraging workbook events can be extremely useful:
Private Sub Workbook_Open()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
With ws
.Tab.Color = RGB(100, 200, 150)
.Cells.Locked = False
.Protect Password:="myPassword"
End With
Next ws
End Sub
These events can automatically set up or alter sheets as soon as the workbook is opened.
Method 5: Sheet-Based Approach with Names

Lastly, you might need to apply different code based on sheet names:
Sub ProcessSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
Select Case ws.Name
Case "Summary"
ws.Cells.EntireColumn.AutoFit
Case "Sales", "Finance"
With ws
.PageSetup.PrintArea = "A1:Z50"
.Cells.Style = "Output"
End With
Case Else
'No action taken
End Select
Next ws
End Sub
This approach gives you granular control, allowing for distinct actions per sheet type.
Having explored these five methods, you're now equipped with various strategies to apply VBA code to multiple sheets in Excel, enhancing your productivity and data management capabilities. From iterating through all sheets, targeting specific ones, to leveraging events, each method serves a unique purpose depending on your requirements. Always remember to consider the following:
- Error handling for unexpected scenarios.
- The impact on workbook performance, especially with large datasets.
- Compatibility with different Excel versions or other Microsoft Office applications.
As you implement these methods, ensure your VBA code is clean, documented, and regularly maintained. Happy VBA coding, and may your spreadsheets always be organized and efficient!
Can I apply VBA code to sheets in a different workbook?
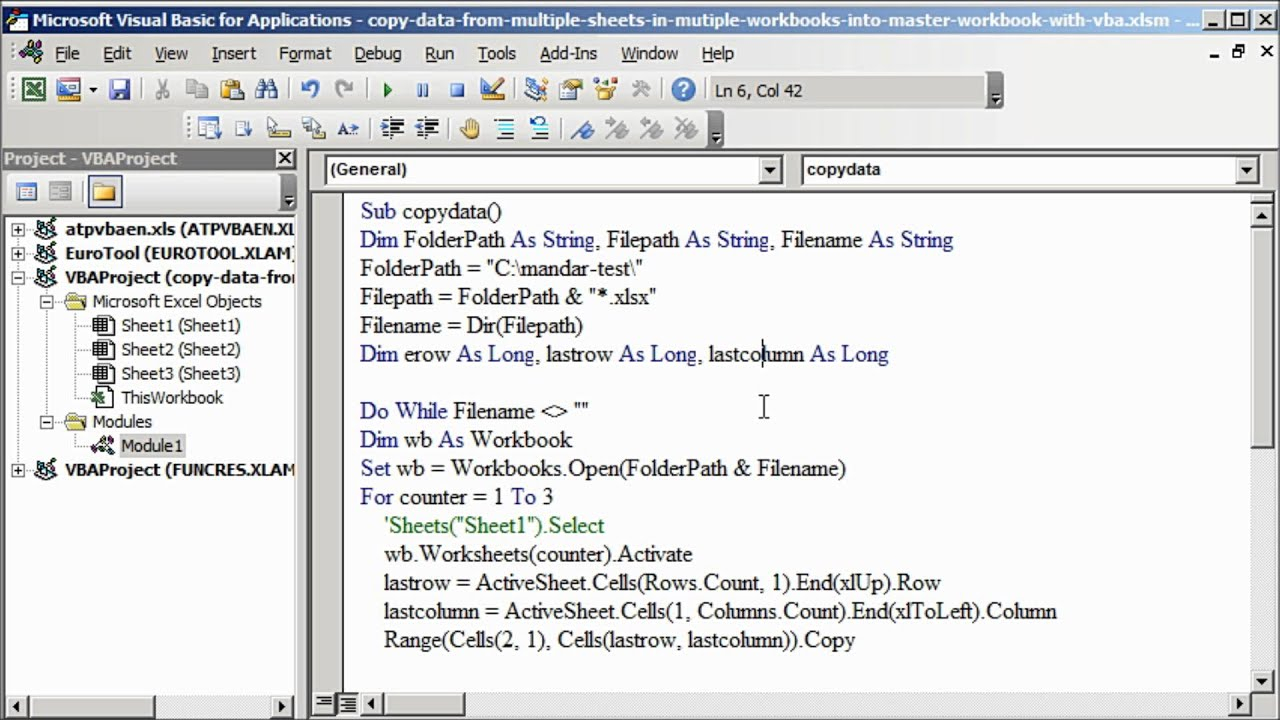
+
Yes, you can modify your VBA code to work with sheets from other open workbooks by referencing those workbooks directly.
Will these methods work with hidden sheets?

+
The methods described will indeed work with hidden sheets, but you might want to make them visible during processing if visibility is crucial for your task.
Is there a performance hit when applying VBA to all sheets?

+
Yes, especially with large datasets or many sheets. Consider optimizing your VBA code or using methods like Workbook-Level Events to minimize the performance impact.
How can I prevent my VBA code from running twice?

+
Use a static boolean variable to check if the code has already run or incorporate a flag within your workbook to control code execution frequency.
Can I use these methods to apply macros from personal.xlsb?

+
Yes, by ensuring your macro in personal.xlsb is accessible, you can call it from any workbook to apply it universally or to specific sheets.